Have you ever wanted to create a simple program in C++, or do you want to create a quiz program so that you can use it in a classroom. Follow this guide and you will be able to create a multiple choice quiz program using C++.
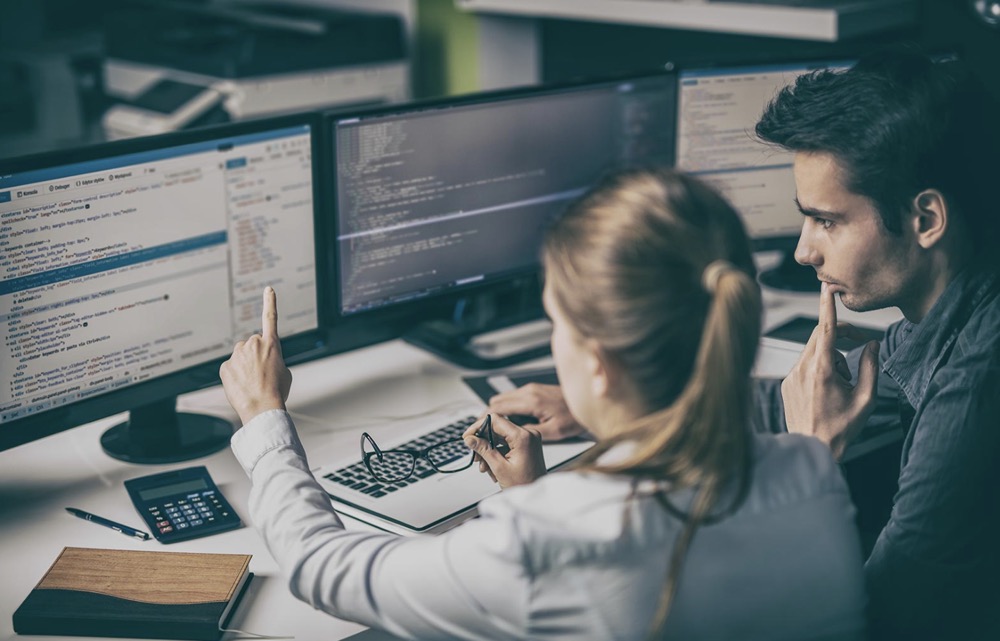
Steps
Start Visual Studio Express and start a new project from the file menu.
In the new project window choose select visual C++ on the right and choose win32 console application on the right.
- In the wizard that shows up next check the box that says empty project.
Add a new .cpp file by going into project and selecting add new item.
Start the file by writing “#include ” and “using namespace std;” at the top of the file.
Add a void function prototype for the end of the program that will take an integer for the number correct.
- A function prototype will make a readily available function header which will be usable for underneath the programs main function, this is required for a void function to work.
- Be sure to use a ‘&’ for the int variable, this will make the variable easier to move to each function.
Add an int function prototype with two parameters, one for a character so that you can send the answer to the function and one for integers so that you can keep track of the amount that the user has right.
- Again be sure to write a ‘&’ for the correct variable in the function.
Write down the main function header for the programs main code.
- This header starts with “int main()” with brackets underneath the code to where the code for the main function will be.
Write the variables for the integer and character for use with the programs main function.
- Be sure to add the integer variable correct to equal 0 so that the program can understand how to use the integer variable correctly.
Write down what you want the answers for the quiz to be using “cout” functions.
- For instance you can type a cout function saying the question’s name..
- You will also use these cout functions for each answer that the user can use.
Write a “cin” line so that the user can type in their answers.
- A “cin” function will let the user write down what the character for the choices in the multiple choice program.
Write down a call for the answer function.
- To call a function you write down the functions name as well as the parameters for example “functionname(answer)”.
- Be sure to write down the correct variables to put into the function so you do not switch them around.
Start writing the new function for the question that the user has answered.
- Be sure to use a switch structure that compares the answer character that the user wrote for there answer.
- Use breaks in each case to not let the program keep going through the switch structure.
- Also be sure to increment the number correct on correct answers so the program knows if the user got the question right.
Return the number correct to the main program.
Repeat the question adding process until you have the desired amount of questions for the quiz.
- Be sure to add the new function prototype for the next question under the first questions function prototype, this will make it so you can write the next questions function right underneath the function for the last question.
Start writing the function for the end of the program.
- Be sure to add the end function in the main function so that you can send the program to the function.
- Make the quiz display how many answers that the user has gotten correct, as well as writing down the percentage that the person has gotten right. Also make sure to use a double integer if you want to make the percentage have a decimal.
Go back to the main function and add “return 0;” to end the program.
- If you want to stop the program from exiting immediately type in “cin.ignore();” two times before the “return 0;” command so that you can exit the program by just hitting enter.
Be sure to test the program to make sure it works.
- If it does not work be sure to look for any errors in the code, Visual Studio Express will show incorrect code with a red square on the scroll line and also by underlining the incorrect code.