Text files can be super useful when coding in Python. You can retrieve information from text files using your code, and you can modify the information that is stored in a text file. In order to be able to do this, it is important to understand how to properly open the file, read the file or write in the file, and then finally save the changes you made (if you made any) to the file. This instruction set will teach you how to do this in a very simple way, and should take no longer than 10-15 minutes! These instructions will specifically discuss how to do this on a MacBook.
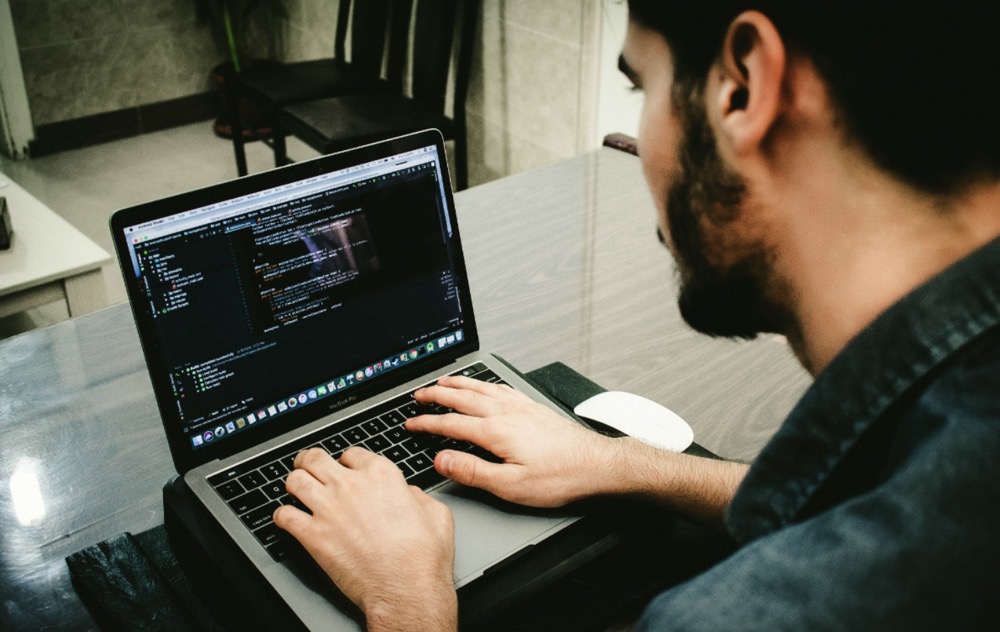
Download Python
Open a browser and navigate to Python.org.
- This should take you to Python’s website where you can download the latest version of Python available.
Click the “Downloads” tab.
- Find where it says “Download the latest version for macOS”.
- Click the yellow button to download the latest version of Python onto your MacBook.WH.shared.addScrollLoadItem(‘cd537fb29a338d8f60dd77126877bddd’)WH.performance.clearMarks(‘image1_rendered’); WH.performance.mark(‘image1_rendered’);
Go to your MacBook’s “Launchpad” and open up “IDLE”.
- This will open up an IDLE Shell where it will output the code that you run. WH.shared.addScrollLoadItem(’11b9fca98097e27a6d02daf6addd154a’)
Click “File” and then “New File” in the upper left-hand corner of your screen
- This will open a blank terminal where you can start typing your Python code.WH.shared.addScrollLoadItem(‘f6a3f3e379d0f87a34255a23560fad13’)”}
Write Your Code
Decide what you would like to create in a Text File.
- For this example, The example below will demonstrate taking each line from a previously created file and printing every other line (every odd line) onto a new file.
Open the text file you would like to use.
- Use any variable you like (example: “oldfile”). Assign this to “open(“YOUR FILE NAME.txt”, ‘r’)”.WH.shared.addScrollLoadItem(‘a3d608df29532d8e7ba5347915297fd6’)
- The letter “r” in this code stands for read.
Assign a new variable for the new file that you are creating
- Use any variable you like different from the first one (example: “newfile”) and assign it to “open(“NEW FILE NAME YOU ARE CREATING.txt”, ‘w’)”.WH.shared.addScrollLoadItem(‘701a1fba4898a4b85ef7480774cc9414’)
- The letter “w” in this code stands for write.
Assign a new variable to read the lines of the file
- Use any new variable (example: “eachline”) and assign it to your original file variable followed by “.readlines()”.WH.shared.addScrollLoadItem(‘1289cb07497fffc015a7d2f2ba7e1767’)
- This is a variable that can now be used to read each line separately in the text file you are reading.
Write the code that you want to implement into the file
- The example contains a for loop that took the location of each line, decided if it was an odd line, and then used the new text file variable “.write(NAME OF FOR LOOP) to write every other line onto the new text file.WH.shared.addScrollLoadItem(‘9faeb4e0a5c1f5fcb3b8932289db81fc’)
Close files you opened
- At the end of your code, write your text file variable “.close()”.
- Do this for each file that you previously opened in your code.WH.shared.addScrollLoadItem(‘568388b736f08dc2c644c4c268e01634’)
- This is an important habit you should have, as it reduces the risk of the file being unwarrantedly modified or read.
Run Your Program
Make sure that every file you used is in the same folder
- This includes the file that your code is written on.
Click “Run” at the top of your screen, and then “Run Module”.
- This is the final step that runs your program,
- Whatever files you wanted to write in should now be created after running this program.WH.shared.addScrollLoadItem(‘0c42a1d8669b6d10c5f79a278fe8c430’)
Tips
- If your code is not working, make sure that all of your files are located in the same folder. This includes your actual code.
- When opening a file, make sure to add “.txt” to the end of the file name.
- Don’t forget to close the quotation marks when opening a file.